Code Generation
Codestral
Codestral is a cutting-edge generative model that has been specifically designed and optimized for code generation tasks, including fill-in-the-middle and code completion. Codestral was trained on 80+ programming languages, enabling it to perform well on both common and less common languages.
We currently offer two domains for Codestral endpoints, both providing FIM and instruct routes:
Domain | Features |
---|---|
codestral.mistral.ai | - Monthly subscription based, currently free to use - Requires a new key for which a phone number is needed |
api.mistral.ai | - Allows you to use your existing API key and you can pay to use Codestral - Ideal for business use |
Wondering which endpoint to use?
- If you're a user, wanting to query Codestral as part of an IDE plugin, codestral.mistral.ai is recommended.
- If you're building a plugin, or anything that exposes these endpoints directly to the user, and expect them to bring their own API keys, you should also target codestral.mistral.ai
- For all other use cases, api.mistral.ai will be better suited
This guide uses api.mistral.ai for demonstration.
This guide will walk you through how to use Codestral fill-in-the-middle endpoint, instruct endpoint, open-weight Codestral model, and several community integrations:
- Fill-in-the-middle endpoint
- Instruct endpoint
- Open-weight Codestral
- Integrations
Fill-in-the-middle endpoint
With this feature, users can define the starting point of the code using a prompt
, and the ending point of the code using an optional suffix
and an optional stop
. The Codestral model will then generate the code that fits in between, making it ideal for tasks that require a specific piece of code to be generated. Below are three examples:
Example 1: Fill in the middle
- python
- curl
import os
from mistralai import Mistral
api_key = os.environ["MISTRAL_API_KEY"]
client = Mistral(api_key=api_key)
model = "codestral-latest"
prompt = "def fibonacci(n: int):"
suffix = "n = int(input('Enter a number: '))\nprint(fibonacci(n))"
response = client.fim.complete(
model=model,
prompt=prompt,
suffix=suffix,
temperature=0,
top_p=1,
)
print(
f"""
{prompt}
{response.choices[0].message.content}
{suffix}
"""
)
curl --location 'https://api.mistral.ai/v1/fim/completions' \
--header 'Content-Type: application/json' \
--header 'Accept: application/json' \
--header "Authorization: Bearer $MISTRAL_API_KEY" \
--data '{
"model": "codestral-latest",
"prompt": "def f(",
"suffix": "return a + b",
"max_tokens": 64,
"temperature": 0
}'
Example 2: Completion
- python
- curl
import os
from mistralai import Mistral
api_key = os.environ["MISTRAL_API_KEY"]
client = Mistral(api_key=api_key)
model = "codestral-latest"
prompt = "def is_odd(n): \n return n % 2 == 1 \ndef test_is_odd():"
response = client.fim.complete(model=model, prompt=prompt, temperature=0, top_p=1)
print(
f"""
{prompt}
{response.choices[0].message.content}
"""
)
curl --location 'https://api.mistral.ai/v1/fim/completions' \
--header 'Content-Type: application/json' \
--header 'Accept: application/json' \
--header "Authorization: Bearer $MISTRAL_API_KEY" \
--data '{
"model": "codestral-latest",
"prompt": "def is_odd(n): \n return n % 2 == 1 \n def test_is_odd():",
"suffix": "",
"max_tokens": 64,
"temperature": 0
}'
Example 3: Stop tokens
We recommend adding stop tokens for IDE autocomplete integrations to prevent the model from being too verbose.
- python
- curl
import os
from mistralai import Mistral
api_key = os.environ["MISTRAL_API_KEY"]
client = Mistral(api_key=api_key)
model = "codestral-latest"
prompt = "def is_odd(n): \n return n % 2 == 1 \ndef test_is_odd():"
suffix = "n = int(input('Enter a number: '))\nprint(fibonacci(n))"
response = client.fim.complete(
model=model, prompt=prompt, suffix=suffix, temperature=0, top_p=1, stop=["\n\n"]
)
print(
f"""
{prompt}
{response.choices[0].message.content}
"""
)
curl --location 'https://api.mistral.ai/v1/fim/completions' \
--header 'Content-Type: application/json' \
--header 'Accept: application/json' \
--header "Authorization: Bearer $MISTRAL_API_KEY" \
--data '{
"model": "codestral-latest",
"prompt": "def is_odd(n): \n return n % 2 == 1 \n def test_is_odd():",
"suffix": "test_is_odd()",
"stop": ["\n\n"],
"max_tokens": 64,
"temperature": 0
}'
Instruct endpoint
We also provide the instruct endpoint of Codestral with the same model codestral-latest
.
The only difference is the endpoint used:
- FIM endpoint: https://api.mistral.ai/v1/fim/completions
- Instruct endpoint: https://api.mistral.ai/v1/chat/completions
- python
- curl
import os
from mistralai import Mistral
api_key = os.environ["MISTRAL_API_KEY"]
client = Mistral(api_key=api_key)
model = "codestral-latest"
message = [{"role": "user", "content": "Write a function for fibonacci"}]
chat_response = client.chat.complete(
model = model,
messages = message
)
print(chat_response.choices[0].message.content)
curl --location "https://api.mistral.ai/v1/chat/completions" \
--header 'Content-Type: application/json' \
--header 'Accept: application/json' \
--header "Authorization: Bearer $MISTRAL_API_KEY" \
--data '{
"model": "codestral-latest",
"messages": [{"role": "user", "content": "Write a function for fibonacci"}]
}'
Codestral Mamba
We have also released Codestral Mamba 7B, a Mamba2 language model specialized in code generation with the instruct endpoint.
- python
- curl
import os
from mistralai import Mistral
api_key = os.environ["MISTRAL_API_KEY"]
client = Mistral(api_key=api_key)
model = "codestral-mamba-latest"
message = [
{
"role": "user",
"content": "Write a function for fibonacci"
}
]
chat_response = client.chat.complete(
model=model,
messages=message
)
print(chat_response.choices[0].message.content)
curl --location "https://api.mistral.ai/v1/chat/completions" \
--header 'Content-Type: application/json' \
--header 'Accept: application/json' \
--header "Authorization: Bearer $MISTRAL_API_KEY" \
--data '{
"model": "codestral-mamba-latest",
"messages": [{"role": "user", "content": "Write a function for fibonacci"}]
}'
Open-weight Codestral and Codestral Mamba
Codestral is available open-weight under the Mistral AI Non-Production (MNPL) License and Codestral Mamba is available open-weight under the Apache 2.0 license.
Check out the README of mistral-inference to learn how to use mistral-inference
to run Codestral.
Integration with continue.dev
Continue.dev supports both Codestral base for code generation and Codestral Instruct for chat.
How to set up Codestral with Continue
Here is a step-by-step guide on how to set up Codestral with Continue using the Mistral AI API:
-
Install the Continue VS Code or JetBrains extension following the instructions here. Please make sure you install Continue version >v0.8.33.
-
Automatic set up:
- Click on the Continue extension iron on the left menu. Select
Mistral API
as a provider, selectCodestral
as a model. - Click "Get API Key" to get Codestral API key.
- Click "Add model", which will automatically populate the config.json.
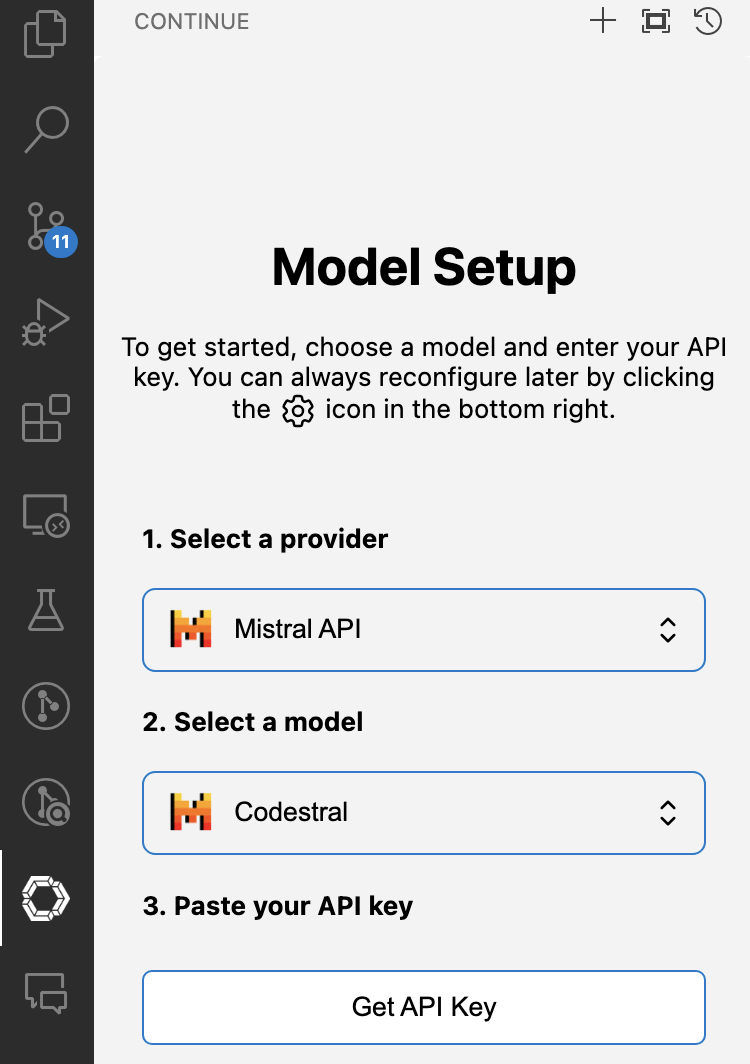
- (alternative) Manually edit config.json
- Click on the gear icon in the bottom right corner of the Continue window to open
~/.continue/config.json
(MacOS) /%userprofile%\.continue\config.json
(Windows) - Log in and request a Codestral API key on Mistral AI's La Plateforme here
- To use Codestral as your model for both
autocomplete
andchat
, replace[API_KEY]
with your Mistral API key below and add it to yourconfig.json
file:
{
"models": [
{
"title": "Codestral",
"provider": "mistral",
"model": "codestral-latest",
"apiKey": "[API_KEY]"
}
],
"tabAutocompleteModel": {
"title": "Codestral",
"provider": "mistral",
"model": "codestral-latest",
"apiKey": "[API_KEY]"
}
}
If you run into any issues or have any questions, please join our Discord and post in #help
channel here
Integration with Tabnine
Tabnine supports Codestral Instruct for chat.
How to set up Codestral with Tabnine
What is Tabnine Chat?
Tabnine Chat is a code-centric chat application that runs in the IDE and allows developers to interact with Tabnine’s AI models in a flexible, free-form way, using natural language. Tabnine Chat also supports dedicated quick actions that use predefined prompts optimized for specific use cases.
Getting started
To start using Tabnine Chat, first launch it in your IDE (VSCode, JetBrains, or Eclipse). Then, learn how to interact with Tabnine Chat, for example, how to ask questions or give instructions. Once you receive your response, you can read, review, and apply it within your code.
Selecting Codestral as Tabnine Chat App model
In the Tabnine Chat App, use the model selector to choose Codestral.
Integration with LangChain
LangChain provides support for Codestral Instruct. Here is how you can use it in LangChain:
# make sure to install `langchain` and `langchain-mistralai` in your Python environment
import os
from langchain_mistralai import ChatMistralAI
from langchain_core.prompts import ChatPromptTemplate
api_key = os.environ["MISTRAL_API_KEY"]
mistral_model = "codestral-latest"
llm = ChatMistralAI(model=mistral_model, temperature=0, api_key=api_key)
llm.invoke([("user", "Write a function for fibonacci")])
For a more complex use case of self-corrective code generation using the instruct Codestral tool use, check out this notebook and this video:
Integration with LlamaIndex
LlamaIndex provides support for Codestral Instruct and Fill In Middle (FIM) endpoints. Here is how you can use it in LlamaIndex:
# make sure to install `llama-index` and `llama-index-llms-mistralai` in your Python enviornment
import os
from llama_index.core.llms import ChatMessage
from llama_index.llms.mistralai import MistralAI
api_key = os.environ["MISTRAL_API_KEY"]
mistral_model = "codestral-latest"
messages = [
ChatMessage(role="user", content="Write a function for fibonacci"),
]
MistralAI(api_key=api_key, model=mistral_model).chat(messages)
Check out more details on using Instruct and Fill In Middle(FIM) with LlamaIndex in this notebook.
Integration with Jupyter AI
Jupyter AI seamlessly integrates Codestral into JupyterLab, offering users a streamlined and enhanced AI-assisted coding experience within the Jupyter ecosystem. This integration boosts productivity and optimizes users' overall interaction with Jupyter.
To get started using Codestral and Jupyter AI in JupyterLab, first install needed packages in your Python environment:
pip install jupyterlab langchain-mistralai jupyter-ai pandas matplotlib
Then launch Jupyter Lab:
jupyter lab
Afterwards, you can select Codestral as your model of choice, input your Mistral API key, and start coding with Codestral!
Integration with JupyterLite
JupyterLite is a project that aims to bring the JupyterLab environment to the web browser, allowing users to run Jupyter directly in their browser without the need for a local installation.
You can try Codestral with JupyterLite in your browser:
Integration with Tabby
Tabby is an open-source AI coding assistant. You can use Codestral for both code completion and chat via Tabby.
To use Codestral in Tabby, configure your model configuration in ~/.tabby/config.toml
as follows.
[model.completion.http]
kind = "mistral/completion"
api_endpoint = "https://api.mistral.ai"
api_key = "secret-api-key"
You can check out Tabby's documentation to learn more.
Integration with E2B
E2B provides open-source secure sandboxes for AI-generated code execution. With E2B, it is easy for developers to add code interpreting capabilities to AI apps using Codestral.
In the following examples, the AI agent performs a data analysis task on an uploaded CSV file, executes the AI-generated code by Codestral in the sandboxed environment by E2B, and returns a chart, saving it as a PNG file.
Python implementation (cookbook):
JS implementation (cookbook):